Quiz: 9 JavaScript Promise Questions
Never drop a promise again!
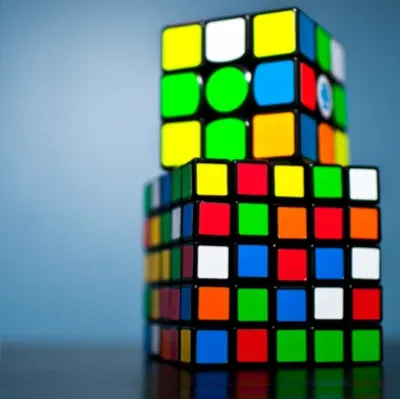
Do you know JavaScript Promises?
- Prove your JavaScript skillz! 🚀
- Check for Hints (Big button, bottom corner).
- Try the code in your browser’s Console (try shortcut
F12
or search it) or use repl.it*. - Please feel free to Tweet at me @justsml. I’d love to hear your thoughts!
👇 Complete 9 Questions Below👇
What will the output be for the following code?
var p = new Promise((resolve, reject) => { reject(Error('The Fails!'))})p.catch(error => console.log(error.message))p.catch(error => console.log(error.message))
We create a Promise using the constructor method, triggering an error immediately with the reject
callback.
Then the .catch
handlers work like the DOM’s .addEventListener(event, callback)
or Event Emitter’s .on(event, callback)
where multiple handler callbacks can be added. Each will be called with the same arguments.
What will the output be for the following code?
var p = new Promise((resolve, reject) => { return Promise.reject(Error('The Fails!'))})p.catch(error => console.log(error.message))p.catch(error => console.log(error.message))
When using the Promise constructor you must invoke either resolve()
or reject()
callbacks. The Promise constructor doesn’t use your return value, so the additional Promise created with Promise.reject()
will effectively never be heard from again.
What will the output be for the following code?
var p = new Promise((resolve, reject) => { reject(Error('The Fails!')) }) .catch(error => console.log(error)) .then(error => console.log(error))
When chaining .then
’s and .catch
’s it is helpful to think of them as a series of steps. Each .then
receives the value returned by the previous .then
(as its argument.) However, if your “step” encountered an error, any subsequent .then
“steps” will be skipped until a .catch
is encountered. If you want to override an error, all you need to do is return a non-error value. It can be accessed via any subsequent .then
.
What will the output be for the following code?
var p = new Promise((resolve, reject) => { reject(Error('The Fails!')) }) .catch(error => console.log(error.message)) .catch(error => console.log(error.message))
When chaining .catch
’s, each one only handles errors thrown in previous .then
or .catch
“steps”. In this example the first .catch
returns the console.log
which could only be accessed via adding a .then()
after both the .catch
’s.
What will the output be for the following code?
new Promise((resolve, reject) => { resolve('Success!') }) .then(() => { throw Error('Oh noes!') }) .catch(error => { return "actually, that worked" }) .catch(error => console.log(error.message))
Hint: .catch
’s can be used to ignore (or override) errors simply by returning a regular value.
This trick works only when there is a subsequent .then
to receive the value.
What will the output be for the following code?
Promise.resolve('Success!') .then(data => { return data.toUpperCase() }) .then(data => { console.log(data) })
Hint: .then
’s pass data sequentially, from return value
to the next .then(value => /* handle value */)
.
A return
is key in order to pass a value to the next .then
.
What will the output be for the following code?
Promise.resolve('Success!') .then(data => { return data.toUpperCase() }) .then(data => { console.log(data) return data }) .then(console.log)
There are 2 console.log
calls which will be called.
What will the output be for the following code?
Promise.resolve('Success!') .then(data => { data.toUpperCase() }) .then(data => { console.log(data) })
Hint: .then
’s pass data sequentially, from return value
to the next .then(value => /* handle value */)
.
A return
is key in order to pass a value to the next .then
.
What will the output be for the following code?
Promise.resolve('Success!') .then(() => { throw Error('Oh noes!') }) .catch(error => { return 'actually, that worked' }) .then(data => { throw Error('The fails!') }) .catch(error => console.log(error.message))