Quiz: Do You Know Modern JavaScript?
Prove your esteemed JavaScript skillz!
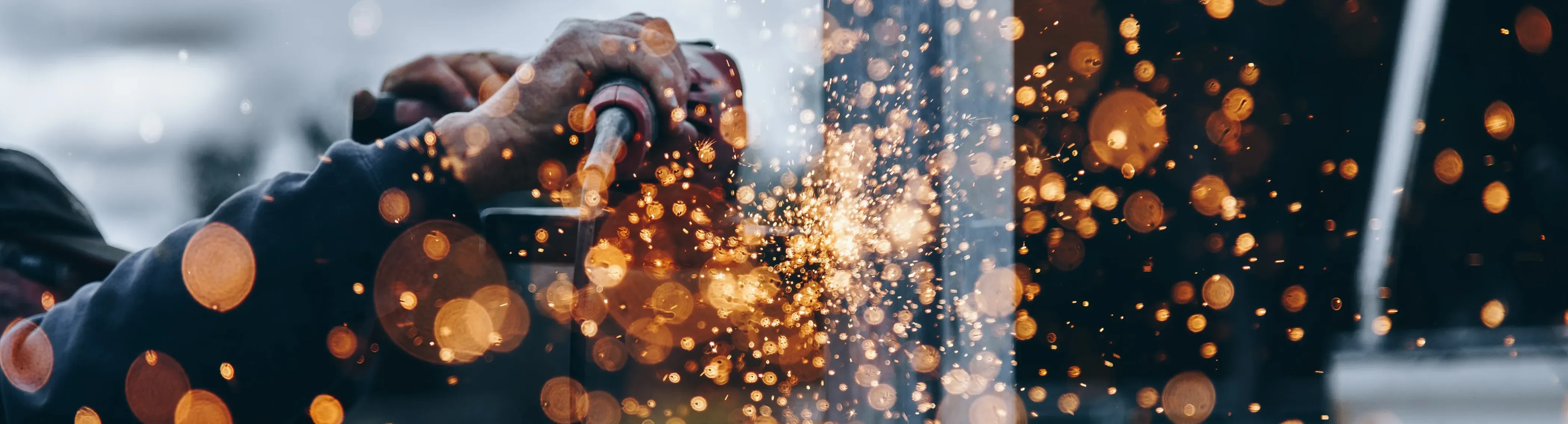
Do you know your ES2015 from ES2022?
- Prove your JavaScript skillz! 🚀
- No login or signup required. ✨
- Multiple choice. 🤖 … How difficult can it be, eh?
What is the output of the following code?
const obj = { foo: null };const result = obj.foo?.bar;console.log(result);
The optional chaining operator (?.
) stops the evaluation if the left-hand side is null
or undefined
. Since obj.foo
is null
, obj.foo?.bar
evaluates to undefined
.
What is the output of the following code?
const a = 42n;const result = a * 2n;console.log(result);
BigInt values are created by appending n
to a number. You cannot mix BigInt with regular numbers in arithmetic operations. Here, both values are BigInt, so the multiplication works, resulting in 84n
.
What does the import()
function return?
const modulePromise = import('./myModule.js');console.log(typeof modulePromise);
The import()
function returns a Promise
that resolves to the module object. In this case, typeof modulePromise
will return 'object'
, as it is an instance of Promise
.
What will be the result of the following code?
const promises = [ Promise.resolve('success'), Promise.reject('error')];Promise.allSettled(promises).then(results => { console.log(results[0].status + ': ' + results[0].value);});
Promise.allSettled
returns an array of objects describing the outcome of each promise. The first promise is fulfilled
with the value 'success'
, so the log statement will print Fulfilled: success
.
What does str.matchAll()
return?
const str = 'foo1bar2baz3';const matches = str.matchAll(/\d/g);console.log(typeof matches);
String.matchAll
returns an iterator of matches, not an array. This iterator can be used to get all the matching groups from a string.
What does import.meta.url
represent?
console.log(import.meta.url);
import.meta
is an object that contains metadata about the current module. The import.meta.url
property represents the URL of the current module, which can be used to get information about where the script is running.
What is the value of a
after the logical assignment?
let a = null;a ||= 10;console.log(a);
The logical OR assignment (||=
) assigns the right-hand value if the left-hand value is falsy (null
, undefined
, 0
, false
, etc.). Since a
is null
, it is assigned the value 10
.
What is the value of b
after the nullish assignment?
let b = null;b ??= 10;console.log(b);
The nullish coalescing assignment (??=
) assigns the right-hand value if the left-hand value is null
or undefined
. Since b
is null
, it is assigned the value 10
.
What does WeakRef
provide?
const obj = { data: 'important' };const ref = new WeakRef(obj);console.log(ref.deref());
WeakRef
provides a weak reference to an object, which allows the object to be garbage collected if no other references exist. The deref()
method returns the original object if it is still available, otherwise undefined
.