Visualizing Promises
Break on through...
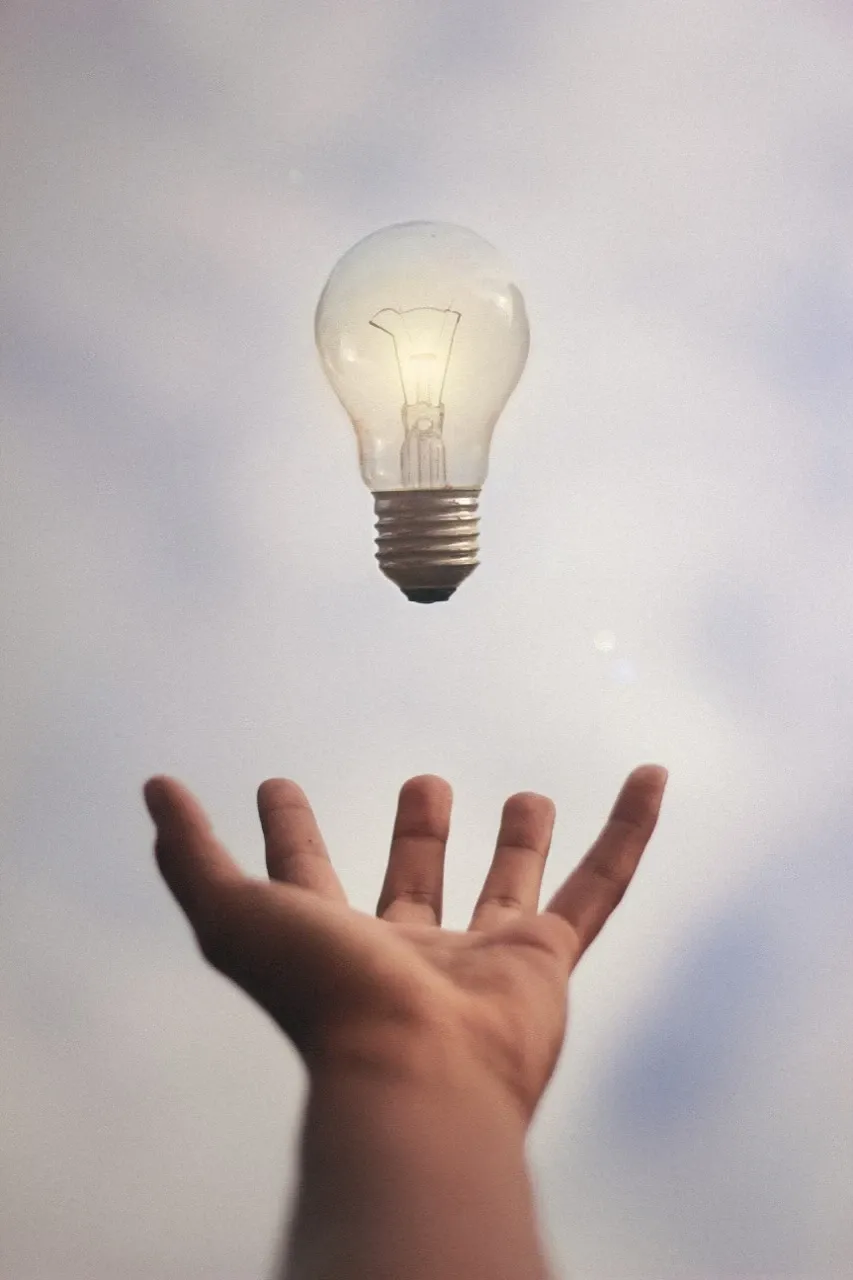
In order to visualize how Promises execute, let’s define a new method delay(millisecs)
.
function delay(millisecs) { return new Promise(resolve => { setTimeout(() => resolve(millisecs), millisecs); });}
This is a utility method which will resolve once the timeout has passed.
The delay in milliseconds will be passed to .then
’s callback.
Let’s look at 4 examples (with animated timelines).
Example #1/4
This shows how console.log()
’s execution will be delayed by delay(msec)
.
delay(1000).then(() => console.log("done"));
Example #2/4
This shows a common mistake.
The console.log
fires right when the delay(1000)
begins. Not after the delay like you probably wanted.
Because console.log
returns undefined
our .then()
is silently ignored.
Note the difference between typeof console.log === 'function'
vs. typeof console.log() === undefined
.
Generally the desired usage for console.log
is shown in Example #1. Make sure you pass functions into .then
and .catch
.
delay(1000).then(console.log("done"));
Example #3/4
3 Promises execute simultaneously.
delay(1000).then(console.log);delay(2000).then(console.log);delay(3000).then(console.log);
Example #4/4
Promise.all
with 3 delay
Promises. They will execute simultaneously.
Promise.all([delay(1000), delay(2000), delay(3000)]).then(console.log);
Credits:
- Animated async diagrams by Patrick Biffle
- Inspiration for this article: https://pouchdb.com/2015/05/18/we-have-a-problem-with-promises.html