Quiz: Data Structures & Algorithms
Can you BS a Binary Tree?
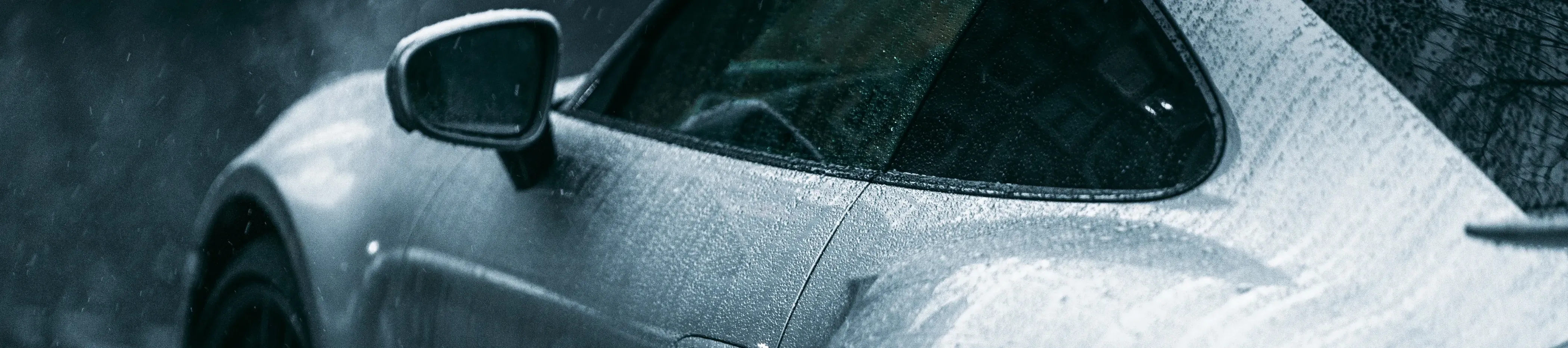
Welcome to my Data Structures and Algorithms quiz!
This quiz will test your knowledge of data structures (Stacks, Lists, Trees, etc), and algorithms (), and time complexity.
20 Questions… Begin!
Which data structure is best suited for a LIFO (Last In, First Out) access pattern?
Stacks are best suited for LIFO access patterns. Queues are best suited for FIFO (First In, First Out) access patterns.
What is the time complexity of an algorithm that always takes the same amount of time to run, regardless of the input size?
O(1) represents constant time complexity. It means the algorithm always takes the same amount of time to run, regardless of the input size.
What is the time complexity for calculating the length of a singly linked list?
To calculate the length of a singly linked list, you must traverse every node from head to tail, resulting in O(n) time complexity.
What is the average time complexity for looking up an element in a balanced Binary Search Tree?
In a balanced BST, the average time complexity for lookup is O(log n) because each level allows the search space to be halved.
What is the time complexity of the Merge Sort algorithm in the worst case?
Merge Sort always operates with a worst-case complexity of O(n log n) as it repeatedly splits the array in half and merges the sorted subarrays.
What is the time complexity of Heap Sort in the worst case?
Heap Sort maintains a worst-case time complexity of O(n log n), as it builds a heap and repeatedly extracts the maximum element.
What is the average time complexity for accessing an element in a hash table?
Hash tables have an average time complexity of O(1) for accessing elements, assuming a good hash function that minimizes collisions.
Which of the following are typical operations performed on a stack?
The primary operations of a stack are Push (add element), Pop (remove element), and Peek (view the top element without removing it).
Which algorithm is commonly used to find the shortest path in a weighted graph with non-negative edges?
Dijkstra’s Algorithm is frequently used for finding the shortest path in graphs with non-negative edge weights. It employs a priority queue to determine the shortest distance efficiently.
Which of the following are examples of balanced tree data structures?
AVL Trees and Red-Black Trees are types of self-balancing trees, which ensure that the tree remains balanced after each insertion or deletion.
What are the two primary operations for a queue?
The two primary operations in a queue are Enqueue (add an element to the back) and Dequeue (remove an element from the front).
What are the conditions for performing topological sorting on a graph?
Topological sorting can be performed on a graph if it is directed and acyclic (DAG). This type of ordering is useful in task scheduling problems.
What is the time complexity of a naive recursive implementation of the Fibonacci series?
The naive recursive implementation of the Fibonacci series has a time complexity of O(2^n) due to the extensive repeated calculations for each Fibonacci number.
Which data structure is commonly used to implement a priority queue?
A priority queue is most often implemented using a heap because it allows efficient extraction of the highest or lowest priority element.
Which of the following are common traversal techniques for a binary tree?
In-order, Pre-order, and Post-order are the three common techniques to traverse binary trees, each with a different order of visiting nodes.
Which of the following properties are true for a min-heap?
In a min-heap, the root is always the smallest element, and the height of the tree is O(log n), making insertion and extraction efficient.
Is the Bubble Sort algorithm stable?
Bubble Sort is a stable sorting algorithm as it preserves the relative order of equal elements during sorting.