Quiz: Symbols & Enumerables
Do you know the less-famous bits of ES2015?
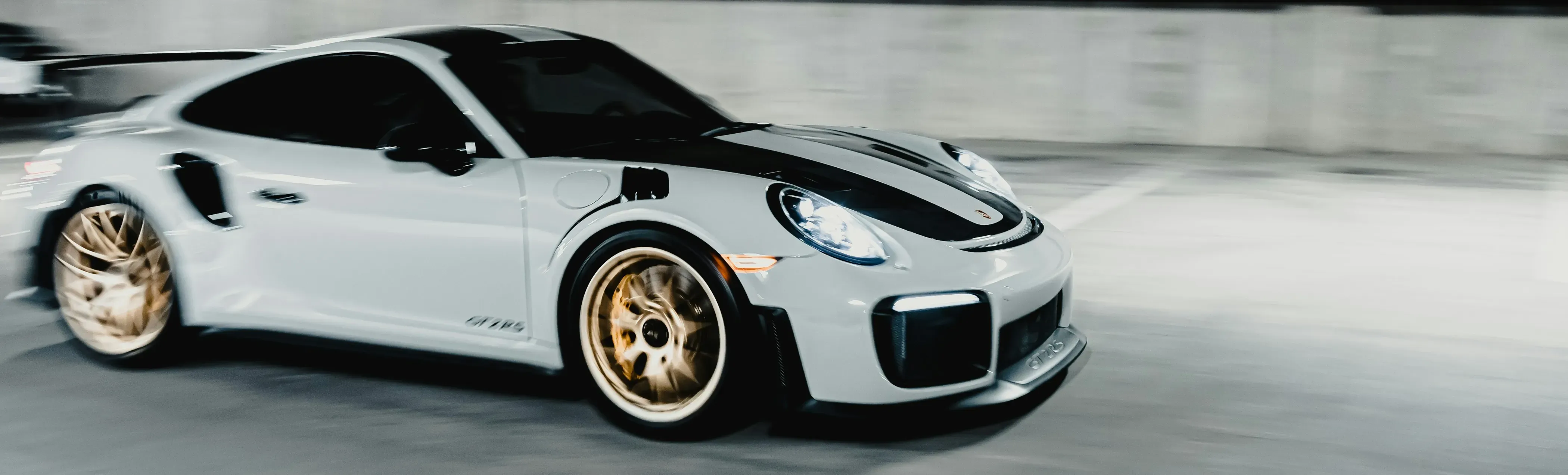
Quiz: JavaScript Interfaces, Symbols, and Enumerables
- Prove your JavaScript skillz! 🚀
- No login or signup required. ✨
- Multiple choice. 🤖 … How difficult can it be, eh?
How should you access a JavaScript object property that uses a getter method?
const obj = { get val() { return 'got it!'; }};console.log(obj.val);
In JavaScript, a getter can be accessed like a normal property. There’s no need to call it like a function.
In this example, accessing obj.val
directly invokes the getter method and outputs got it!
.
What is the correct way to create a truly unique property key for a JavaScript object?
const uniqueKey = Symbol('myUniqueKey');const obj = { [uniqueKey]: 'unique value'};console.log(obj[uniqueKey]);
Symbols are a unique and immutable primitive type that can be used as keys for object properties. This helps in avoiding name collisions, especially in large codebases or when writing reusable libraries.
Will the property age
be listed during a for...in
iteration?
const person = {};Object.defineProperty(person, 'age', { value: 25, enumerable: true});for (let key in person) { console.log(key);}
The enumerable
property in Object.defineProperty()
controls whether the property will show up in enumeration methods like for...in
. In this example, since enumerable: true
, the age
property will be listed during iteration.
What is the default enumerability of a property when using Object.defineProperty()
without specifying enumerable
?
const car = {};Object.defineProperty(car, 'make', { value: 'Toyota'});console.log(Object.keys(car));
When you use Object.defineProperty()
without specifying enumerable
, its default value is false
. This means the make
property will not show up in Object.keys()
or other enumeration methods.
What will be the result of the following comparison?
const sym1 = Symbol('id');const sym2 = Symbol('id');console.log(sym1 === sym2);
Each call to Symbol()
creates a unique and immutable value, even if the description is the same. In this case, sym1
and sym2
are different symbols, so the comparison returns false
.
Will the Symbol-keyed property be listed during a for...in
iteration?
const sym = Symbol('uniqueKey');const obj = { [sym]: 'symbol value', regularKey: 'regular value'};for (let key in obj) { console.log(key);}
Properties keyed by Symbols are not enumerable in for...in
loops or Object.keys()
. In this example, only regularKey
will be listed, not the Symbol-keyed property.
Which method can be used to retrieve all Symbol keys of an object?
The Object.getOwnPropertySymbols()
method is used to retrieve all Symbol properties of an object.
const sym1 = Symbol('id');const sym2 = Symbol('name');const obj = { [sym1]: 'symbol value', [sym2]: 'another symbol value'};console.log(Object.getOwnPropertySymbols(obj));// [Symbol(id), Symbol(name)]