Quiz: Do You know Modern CSS? (for 2025)
Are you front-end enough?
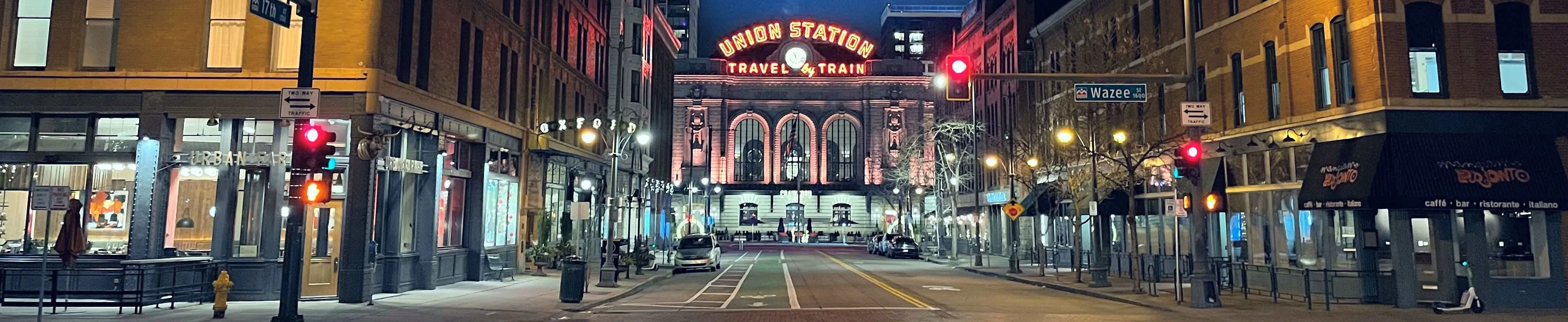
Quiz: Do You Know CSS?
- Modern CSS? 🤔
- Does CSS belong on your resume??? 🚀
- Multiple choice. 🤖 … How difficult can it be, eh?
What is the correct way to use a CSS variable called --main-color
to set an element’s background color?
:root { --main-color: blue;}div { /* How do we use --main-color here? */}
CSS variables are used with the var
function, so the correct answer is background-color: var(--main-color);
. This syntax retrieves the value of --main-color
and applies it.
The other options may be familiar from other languages or preprocessor syntaxes, namely Sass or Less.
If the viewport width is 400px, what will the computed width of the following element be?
div { width: min(250px, 50%);}
The min()
function will choose the smallest value between 250px and 50% of its parents width.
To understand the computed value, we need to convert the relative units to pixels:
50%
of400px
is200px
250px
is already in pixels
/* This gets computed to */width: min(250px, 200px);/* -> 200px wins */
The min()
function is especially useful for responsive design, where you can ensure a component (or font size) doesn’t exceed a certain limit.
Given a container with a width of 200px, what would be the computed width of the <div>
?
div { width: max(50px, 10%, 6rem);}
The max()
function accepts 2 or more inputs, and will use the largest value automatically. In this case, the width works out to 96px
.
To understand the computed value, we need to convert the relative units to pixels:
50px
is already in pixels10%
of200px
is20px
6rem
is6 * 16px
(the default font size) which is96px
/* This gets computed to */width: max(50px, 20px, 96px);/* -> 96px wins */
What is the effect of using minmax(100px, 200px)
for a CSS grid track?
grid-template-columns: minmax(100px, 200px);
Using minmax(100px, 200px)
allows the grid track to resize between 100px
and 200px
, adapting to the available space but never going below 100px
or above 200px
.
You can create auto-adjusting layouts where the container and children each play a role in computing layouts. This is powerful when combined with repeat()
and auto-fill
or auto-fit
, which will create as many tracks as possible within the constraints.
What color will the background be for the following CSS?
div { background: var(--primary, olivedrab);}
The var()
function allows you to set a fallback value if the variable is not defined. In this case, the background will be red
because --primary
is not defined.
This is a great way to ensure your styles don’t break if a variable is missing or not supported.
What does the clamp()
do?
.card { width: clamp(200px, 50vw, 500px);}
The clamp()
function allows the width to scale based on 10vw
, but keeps it within a range of 200px to 600px.
This means the width will be 200px when the viewport is less than 2000px, and 600px when the viewport is greater than 6000px. In between, it will scale linearly.
It lets you be auto-magically responsive! The thing to know about clamp
is it combines fixed units with responsive or computed units.
Normally you wouldn’t want to use viewport units for font sizes, but with clamp()
we can ensure the font size doesn’t get too small or too large.
Does CSS support nesting natively?
Yes! We finally have native CSS nesting! CSS introduced native nesting syntax in recent years (2023), allowing for hierarchical styling directly in CSS.
Is this a correct use of native CSS nesting?
.container { color: black; .title { color: white; background: black; }}
The .title
class is nested within the .container
class, and the properties are applied as expected.
This is a great way to keep related styles together and avoid long selectors.
What background color will be applied to direct child div
s of .container
?
.container { background-color: red; > div { background-color: white; } background-color: blue !important;}
The >
selector in the nested rule applies background-color: yellow
only to direct children div
elements within .container
.
The last rule, background-color: blue !important;
, is a little distraction. It’s outside the nested rule and will be applied to all .container
elements.
How can you change the value of a CSS variable at runtime?
CSS variables can be set using classes & JavaScript. They can even be defined ‘after’ they are technically used.
document.documentElement.style.setProperty('--main-color', 'blue');
This will change the value of --main-color
to blue
for the entire document.
CSS variables are mutable, and can be changed at runtime using JavaScript.
They also can be changed by adding or removing classes, which is a common pattern for theming.
What will be the computed width of the element?
:root { --base-width: 100px;}div { width: calc(var(--base-width) + 10px);}
The calc()
function combines the value of --base-width
(100px) with an additional 10px, resulting in a width of 110px.