Quiz: 14 JavaScript Date Questions
Learn to impress at parties with JS trivia! ✨
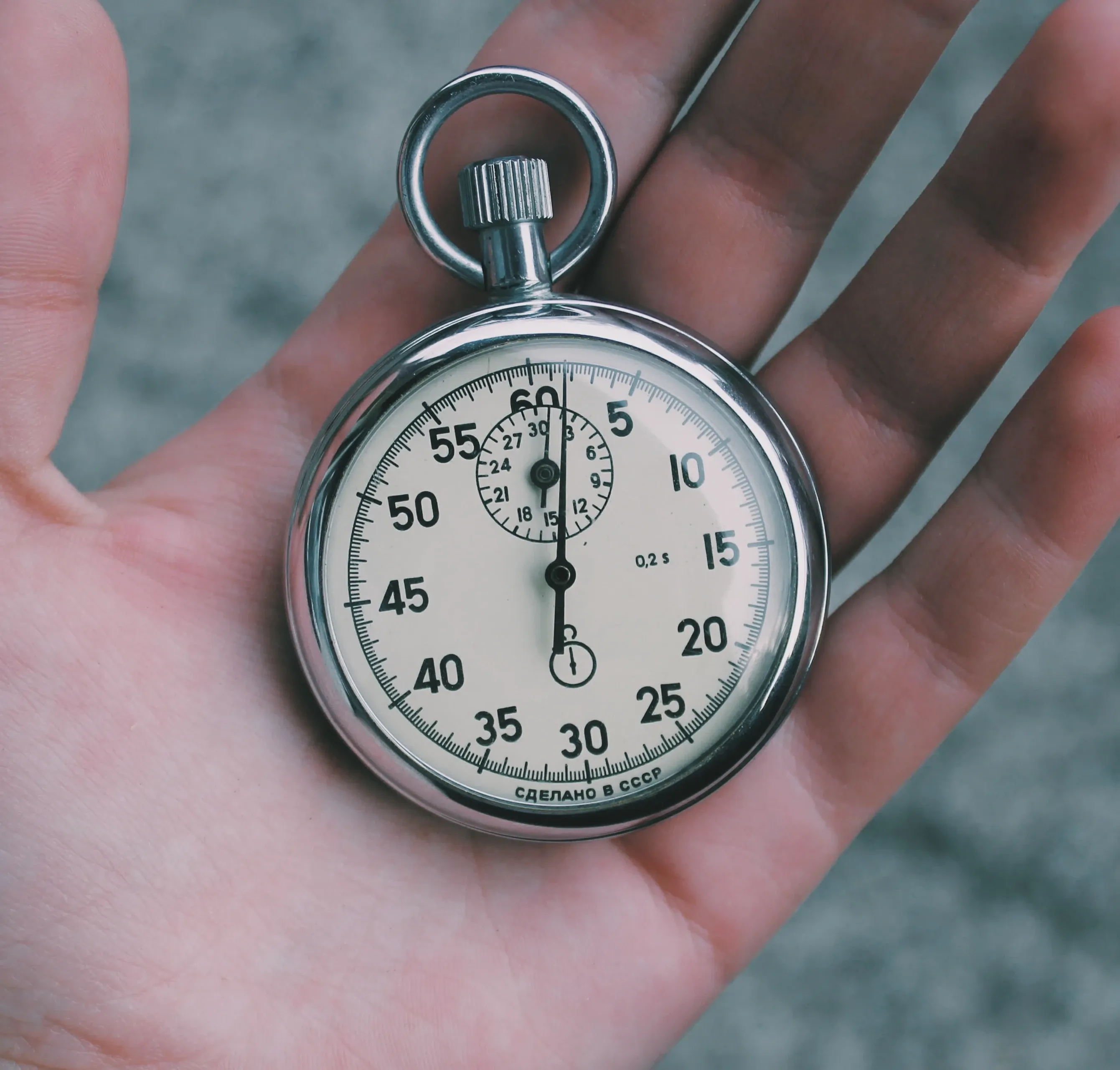
How well do you know the Date
class?
- Prove your JavaScript skillz! 🚀
- No login or signup required. ✨
- Multiple choice. 🤖 … How difficult can it be, eh?
Outline
The Date
class in JavaScript has a notoriously difficult API. It was inherited from Java, and I can only assume was inspired ancient neolithic time keeping methods.
The struggle to get Date
’s leads many developers to use 3rd party libraries without question. While often a safe and reliable choice, these libraries are rarely needed for formatting dates or localization!
This quiz is designed to test (and deepen) your knowledge of the native Date
API. Use the green buttons for hints & explanations! Hopefully by the end of the challenge you’ll have solidified your understanding of Date
’s in JavaScript.
NOTE: Assume all examples assume GMT-7 local timezone.
👇 14 Questions Below 👇
What will the output include?
const d1 = new Date(2020, 1, 1)console.log(d1)
The Month argument is zero-based. With a range of 0-11 (using western calendars.)
‘February’ has an index value of one. (Think of it like an array lookup.)
What will the output include?
const d2 = new Date(2020, 0, 1)console.log(d2)
The Month argument is zero-based. With a range of 0-11 (using western calendars.)
‘January’ has an index value of zero. (Think of it like an array lookup.)
What will the output include?
const d3 = Date('Thu, 01 Jan 1970 00:00:00 GMT')console.log(d3)
Don’t forget the new
keyword! Date
is a class, and should be called with new
.
Date('...')
without new
ignores what you give it. It appears to always produce the current date & time with new Date()
(no arguments).
This is a common gotcha that’s easy to overlook, even in code review.
What will the output include?
const date = new Date(2020)console.log(date.getFullYear())
A Date instance created with a single integer argument is interpreted as a Unix Epoch
value. Epoch
is a count of milliseconds since Jan 1st, 1970.
A value of 2020
(milliseconds) translates to 2 seconds after Jan 1st, 1970.
Then since our local time zone is a negative offset of -7 hours, we end up with Wed Dec 31 1969 17:00:02 GMT-0700 (Mountain Standard Time)
.
You can side-step the local timezone offset by using .getUTCFullYear()
.
What value will print to the console?
const d1 = new Date('2020-01-01')const d2 = new Date('2020-01-01T00:00')console.log(d1.getFullYear(), d2.getFullYear())
The string without a T
time value may appear to be Jan 1st, 2020 - but it’s interpreted as a GMT time, and when adjusted to our local timezone (GMT-7) we find we’re still in 2019.
The Date
class implicitly applies local time to date strings when a time is not provided.
The T00:00
causes it to adjust for local time.
The first date is interpreted as Tue Dec 31 2019 17:00:00 GMT-0700 (Mountain Standard Time)
.
The second date is interpreted as Wed Jan 01 2020 00:00:00 GMT-0700 (Mountain Standard Time)
.
Select an incorrect formatting method:
The method toLocaleFormat()
isn’t standard! It may look familiar since it’s from an ancient 3rd party library.
Check out the toLocaleDateString
docs method. It’s behavior is documented under Intl.DateTimeFormat
.
What will the output include?
var date = Date.UTC('2020-01-02T00:00')console.log(date.toUTCString())
You’ll get TypeError: date.toUTCString is not a function
, since Date.UTC()
returns an integer in milliseconds, not a date instance.
What will the output include?
const d = Date.UTC(2020, 0, 1)console.log(d)
The helper method Date.UTC
doesn’t return a date instance. It returns an integer in milliseconds.
What will the output include?
// Assume local TZ is -07:00const d = new Date(Date.UTC(2020, 0, 1))console.log(d.getTimezoneOffset())
Date’s will be implicitly presented in local time, with an (effectively) unchanging .getTimezoneOffset()
.
Date
instances do not store timezone data. They store the # of milliseconds since the Unix Epoch (Jan 1, 1970). The timezone is accounted for when Date String parsing & rendering. The default display behavior is automatically determined based on the system or browser’s locale settings.
What will the output include?
const d = new Date(2020, 0, 1)d.setDate(1)console.log(d)
The .setDate()
method sets the day of the month, based on the given instance’s current month.
If a value is provided outside of the number of days available, the date instance month value will be adjusted (e.g. A setDate(32)
in January will calculate as February 1st.)
What will the output include?
const d = new Date(2020, 0, 1)d.setMonth(1)console.log(d)
The .setMonth()
method sets the month of the given date instance.
The month argument is zero-based, with a range of 0-11 (using western calendars.)
What will the output include?
const d = new Date(2020, 0, 1)d.setMonth(12)console.log(d)
The .setMonth()
method sets the month of the given date instance.
The month
argument is zero-based, with 12 values in the range of 0-11 (using western calendars.)
Here we see the year is adjusted to 2021, because setMonth(12)
is 1 more than 11 (December).
What will the output include?
const d = new Date(2020, 0, 1)d.setMonth(13)console.log(d)
The .setMonth()
method sets the month of the given date instance.
The month argument is zero-based, with a range of 0-11 (using western calendars.)
Here we see the month and year is adjusted to February 2021, because setMonth(13)
is 2 more than 11 (December).
What will the output include?
const d = new Date(2020, 0, 1)d.setMonth(-1)console.log(d)
The .setMonth()
method sets the month of the given date instance.
The month argument is zero-based, with a range of 0-11 (using western calendars.)
Here we see the month and year rolls back to December 2019, because setMonth(-1)
is less than 0 (January).